Unity SCORM Course Generator
Converting MindForge Interactive Training into the SCORM Format
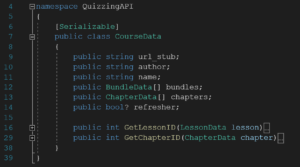
For several years, MindForge courses were only available on their proprietary apps and website, and could not be shared anywhere else. This prevented MindForge from expanding their reach since most of the e-learning world runs on the Sharable Content Object Reference Model (SCORM) format. I undertook a project to create a generator that would allow our Unity projects to be loaded and communicate with a SCORM-compliant LMS. The generator is another Unity project that links to a course’s scripts through the package manager and loads the course’s assets from assetbundles.
MindForge normally stores course assetbundles on Amazon S3 and retrieves only the bundles required for a user’s training when they launch the course. The distribution partners we worked with requested that all assets exist as part of the SCORM package and not be downloaded on the fly, so I added the course bundles to the StreamingAssets folder and loaded them at runtime from there. StreamingAssets on WebGL are accessed through a web request, but it isn’t a cross-origin request and is usually quite fast.
I also placed the video assets for a course in StreamingAssets since the video player we already had can access them easily from there. A MindForge course is defined by a series of JSON files, including basic course information such as lesson structure, requirements, quizzes and quiz questions, and so on. I added these JSON files as TextAssets and referenced them in a custom ScriptableObject called SCORM_CourseResources, which also includes references to any static image referenced in and of the course’s quizzes. The videos, bundles, and SCORM_CourseResources completely define a course and all of its content.
After gathering all of those assets and defining how they are accessed, the only tasks left were to create a player that could run through the course’s structure and show everything to a user in order. I used three main scripts for this purpose: SCORM_CourseController, SCORM_MainSceneController, and CourseLifecycleEvents. CourseLifecycleEvents doesn’t get the SCORM prefix because it can be used in any context. The main scene controller handles the UI for the quiz system and the video player. The course controller handles the overall flow of the course and communication with the LMS. The lifecycle events class is a simple singleton consisting of several public events that any script can subscribe to or invoke, forming a basic messaging system and decouples the course controller and scene controller from each other and the rest of the course logic.
This project was a major success. With just a few days of work, I was able to unlock MindForge’s courses from their self-imposed prison and allowed them to be distributed in any e-learning marketplace using a SCORM-compliant LMS. The most difficult part of the project was removing our courses’ reliance on MindForge servers and networking code, requiring some refactoring of very old code.
Scripts
CourseLifecycleEvents.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | #if SCORM using MindForge.Common.Util; using QuizzingAPI; using System; /// <summary> /// Initially created for SCORM course conversion. Might be useful in the future too. /// </summary> public class CourseLifecycleEvents : Singleton<CourseLifecycleEvents> { public Action courseStarted; public Action userReadyToStart; public Action<QuizData> quizStarted; public Action<string, string> videoStarted; // (videoPath,subtitlePath) public Action videoComplete; public Action lessonCompleted; public Action quizCompleted; public Action simCompleted; public Action lessonQuit; public Action mainSceneLoaded; public Action bundlesLoadStarted; public Action<float> bundlesLoadProgress; // % complete of total bundle loading public Action courseCompleted; } #endif |
SCORM_CourseController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 | using MindForge.Common.Managers; using QuizzingAPI; using Scorm; using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Threading.Tasks; using UnityEngine; using UnityEngine.Networking; using UnityEngine.SceneManagement; public class SCORM_CourseController : MonoBehaviour, ICourseImageProvider { public static SCORM_CourseController Instance { get; private set; } [SerializeField] private SCORM_CourseResources courseInfo; [SerializeField] private TextAsset UILocalizationFile; private IScormService scormService; private CourseData courseData; private int chapterIndex = 0; private int lessonIndex = 0; private int scormLessonsCompleted = 0; private void Awake() { if(Instance != null) { Destroy(gameObject); return; } Instance = this; DontDestroyOnLoad(gameObject); LocalizationText.SetLanguage("EN"); LocalizationText.AddToLibrary(UILocalizationFile); courseData = JsonUtility.FromJson<CourseData>(courseInfo.applicationJSON.text); #if UNITY_EDITOR scormService = new ScormPlayerPrefsService(); #else scormService = new ScormService(); #endif if(scormService.Initialize(Scorm.Version.Scorm_2004)) // Starts comms with the LMS { scormService.SetMinScore(0); scormService.SetMaxScore(GetLessonCount()); scormService.SetRawScore(0); if (scormService.GetLessonStatus() != LessonStatus.Completed) { scormService.SetLessonStatus(LessonStatus.Incomplete); } scormService.Commit(); } CourseLifecycleEvents.Instance.lessonCompleted += AdvanceLesson; CourseLifecycleEvents.Instance.userReadyToStart += StartLesson; CourseLifecycleEvents.Instance.mainSceneLoaded += StartLesson; CourseLifecycleEvents.Instance.simCompleted += OnSimCompleted; CourseLifecycleEvents.Instance.quizCompleted += StartLesson; CourseLifecycleEvents.Instance.videoComplete += StartLesson; CourseLifecycleEvents.Instance.lessonCompleted += SCORM_OnLessonComplete; CourseLifecycleEvents.Instance.courseCompleted += SCORM_OnCourseComplete; } public Sprite GetCourseImage(string image) { string imageName = Path.GetFileNameWithoutExtension(image); for(int i = 0; i < courseInfo.images.Length; i++) { if (courseInfo.images[i].name == imageName) return courseInfo.images[i]; } Debug.LogError($"Couldn't find sprite \"{image}\" in course resources!"); return null; } public void GetCourseImageAsync(string image, Action<Sprite> result) { // Not async, but it doesn't need to be yet result?.Invoke(GetCourseImage(image)); } private void StartLesson() { if (chapterIndex >= courseData.chapters.Length) return; // reached the end of the course LessonData lesson = courseData.chapters[chapterIndex].lessons[lessonIndex]; if (lesson.content.Length > 1) Debug.LogError($"More than one piece of content:{courseData.chapters[chapterIndex].name}, {lesson.name}", this); ContentData content = lesson.content[0]; switch(content.type) { case "quiz": Debug.Log($"Starting quiz:({lesson.name},{content.name})", this); StartQuiz(content); break; case "unity": case "scene": Debug.Log($"Starting sim: ({lesson.name}, {content.name})", this); StartSim(content); break; case "video": Debug.Log($"Starting video: ({lesson.name}, {content.name})", this); StartVideo(content); break; default: Debug.LogError($"Unknown content type:{content.type}, ({lesson.name},{content.name}), (ch:{chapterIndex},l:{lessonIndex}", this); CourseLifecycleEvents.Instance.lessonCompleted?.Invoke(); CourseLifecycleEvents.Instance.courseStarted?.Invoke(); break; } } private void AdvanceLesson() { lessonIndex++; if(lessonIndex >= courseData.chapters[chapterIndex].lessons.Length) { chapterIndex++; lessonIndex = 0; } if(chapterIndex >= courseData.chapters.Length) { // finished all content, end the course Debug.Log("Content done, good job!", this); CourseLifecycleEvents.Instance.courseCompleted?.Invoke(); } } private QuizData GetQuizFromContent(ContentData content) { QuizData quizData = null; for (int i = 0; i < courseInfo.quizzesJSON.Length; i++) { quizData = JsonUtility.FromJson<QuizData>(courseInfo.quizzesJSON[i].text); if (quizData.quiz_name == content.name) break; quizData = null; } if (quizData == null) { Debug.LogError($"Quiz data not found: {content.name}"); return null; } // Populate question data from courseInfo JSON quizData.QuizQuestions = new QuestionData[quizData.questions.Length]; for(int i = 0; i < quizData.questions.Length; i++) { for(int j = 0; j < courseInfo.questionsJSON.Length; j++) { QuestionData questionData = JsonUtility.FromJson<QuestionData>(courseInfo.questionsJSON[j].text); if(questionData.question_name == quizData.questions[i]) { quizData.QuizQuestions[i] = JsonUtility.FromJson(courseInfo.questionsJSON[j].text, questionData.Type) as QuestionData; break; } } } return quizData; } private void StartQuiz(ContentData content) { QuizData quizData = GetQuizFromContent(content); if(quizData == null) { Debug.LogError($"Couldn't find quiz data:{content.name}", this); return; } CourseLifecycleEvents.Instance.quizStarted?.Invoke(quizData); } private void StartSim(ContentData content) { StartCoroutine(StartSimRoutine(content)); } private IEnumerator StartSimRoutine(ContentData contentData) { CourseLifecycleEvents.Instance.bundlesLoadStarted?.Invoke(); float progressPerBundle = 1.0f / courseData.bundles.Length; // Lazy route: just load all the bundles // TODO: fix this for larger sims like PFAS for (int i = 0; i < courseData.bundles.Length; i++) { if (AssetBundleManager.Instance.IsBundleLoaded(courseData.bundles[i].name)) continue; #if UNITY_EDITOR AssetBundleCreateRequest req = AssetBundle.LoadFromFileAsync(Path.Combine(Application.streamingAssetsPath, courseData.bundles[i].name)); while(!req.isDone) { yield return null; CourseLifecycleEvents.Instance.bundlesLoadProgress?.Invoke(i*progressPerBundle + req.progress * progressPerBundle); } AssetBundle bundle = req.assetBundle; #else // Can't load from file on webGL UnityWebRequest req = UnityWebRequest.Get(Path.Combine(Application.streamingAssetsPath, courseData.bundles[i].name)); var webRequestOp = req.SendWebRequest(); while (!webRequestOp.isDone) { yield return null; // Downloading is 80% the progress of the bundle CourseLifecycleEvents.Instance.bundlesLoadProgress?.Invoke( progressPerBundle * (i + 0.8f * webRequestOp.progress)); } var bundleLoadOp = AssetBundle.LoadFromMemoryAsync(req.downloadHandler.data); while(!bundleLoadOp.isDone) { yield return null; CourseLifecycleEvents.Instance.bundlesLoadProgress?.Invoke( progressPerBundle * (i + 0.8f + 0.2f * bundleLoadOp.progress)); } AssetBundle bundle = bundleLoadOp.assetBundle; #endif if (bundle == null) { Debug.LogError($"Failed to load bundle: {courseData.bundles[i].name}", this); continue; } Debug.Log($"Bundle loaded: {courseData.bundles[i].name}", this); AssetBundleManager.Instance.AddLoadedBundle(courseData.bundles[i].name, bundle); } SceneManager.LoadScene(contentData.name); } private void StartVideo(ContentData content) { CourseLifecycleEvents.Instance.videoStarted?.Invoke( Path.Combine(Application.streamingAssetsPath, content.name + ".mp4"), Path.Combine(Application.streamingAssetsPath, content.name + ".srt")); } private void OnSimCompleted() { Debug.Log("Sim complete"); SceneManager.LoadScene("SCORM Main"); } private int GetLessonCount() { int count = 0; for(int i = 0; i < courseData.chapters.Length; i++) { count += courseData.chapters[i].lessons.Length; } return count; } private void SCORM_OnLessonComplete() { scormLessonsCompleted++; scormService.SetRawScore(scormLessonsCompleted); scormService.Commit(); } private void SCORM_OnCourseComplete() { scormService.SetLessonStatus(LessonStatus.Completed); scormService.Commit(); scormService.Finish(); } } |
SCORM_CourseResources.cs
1 2 3 4 5 6 7 8 9 10 | using UnityEngine; [CreateAssetMenu] public class SCORM_CourseResources : ScriptableObject { [SerializeField] public TextAsset applicationJSON; [SerializeField] public TextAsset[] quizzesJSON; [SerializeField] public TextAsset[] questionsJSON; [SerializeField] public Sprite[] images; } |
SCORM_MainSceneController.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 | using QuizzingAPI; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class SCORM_MainSceneController : MonoBehaviour { [SerializeField] private GameObject quizBaseUI; [SerializeField] private QuizController quizController; [SerializeField] private GameObject videoBaseUI; [SerializeField] private VideoPlayer videoPlayer; [SerializeField] private GameObject loadingScreenCanvas; [SerializeField] private Image loadingImage; [SerializeField] private GameObject startScreenUI; [SerializeField] private Button startScreenButton; [SerializeField] private GameObject endScreenUI; private static bool firstLoad = true; // Start is called before the first frame update private void Start() { CourseLifecycleEvents.Instance.quizStarted += OnQuizStart; CourseLifecycleEvents.Instance.videoStarted += OnVideoStart; CourseLifecycleEvents.Instance.bundlesLoadStarted += OnBundlesLoadStart; CourseLifecycleEvents.Instance.bundlesLoadProgress += OnBundlesLoadProgress; CourseLifecycleEvents.Instance.courseStarted += OnCourseStarted; CourseLifecycleEvents.Instance.courseCompleted += OnCourseCompleted; startScreenButton.onClick.AddListener(OnStartButtonClicked); quizController.OnComplete += OnQuizComplete; videoPlayer.onComplete.AddListener(OnVideoCompleted); if(firstLoad) { firstLoad = false; CourseLifecycleEvents.Instance.courseStarted?.Invoke(); } else { CourseLifecycleEvents.Instance.mainSceneLoaded?.Invoke(); } } private void OnDestroy() { if (CourseLifecycleEvents.Instance == null) return; // Application is unloading, so instances may be null CourseLifecycleEvents.Instance.quizStarted -= OnQuizStart; CourseLifecycleEvents.Instance.videoStarted -= OnVideoStart; CourseLifecycleEvents.Instance.bundlesLoadStarted -= OnBundlesLoadStart; CourseLifecycleEvents.Instance.bundlesLoadProgress -= OnBundlesLoadProgress; CourseLifecycleEvents.Instance.courseStarted -= OnCourseStarted; CourseLifecycleEvents.Instance.courseCompleted -= OnCourseCompleted; } private void OnQuizStart(QuizData quizData) { quizBaseUI.SetActive(true); quizController.SetupQuiz(quizData, SCORM_CourseController.Instance); } private void OnQuizComplete(int correct, int total) { Debug.Log("Quiz complete"); quizBaseUI.SetActive(false); CourseLifecycleEvents.Instance.lessonCompleted?.Invoke(); CourseLifecycleEvents.Instance.quizCompleted?.Invoke(); } private void OnVideoStart(string videoPath, string subtitlesPath) { videoBaseUI.SetActive(true); videoPlayer.SetVideo(videoPath, subtitlesPath); } private void OnVideoCompleted() { Debug.Log("Video complete"); videoBaseUI.SetActive(false); CourseLifecycleEvents.Instance.lessonCompleted?.Invoke(); CourseLifecycleEvents.Instance.videoComplete?.Invoke(); } private void OnBundlesLoadStart() { loadingScreenCanvas.SetActive(true); } private void OnBundlesLoadProgress(float progress) { loadingImage.fillAmount = progress; } private void OnCourseStarted() { startScreenUI.SetActive(true); } private void OnStartButtonClicked() { CourseLifecycleEvents.Instance.userReadyToStart?.Invoke(); } private void OnCourseCompleted() { endScreenUI.SetActive(true); } } |